Here you can see API endpoints related to package purchases.
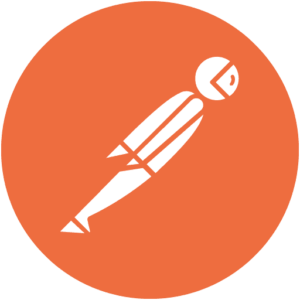
You can see all the API endpoints with examples of requests and responses in the Amelia API Postman collection which you can download here.
Authorization
All Amelia endpoints use an API key authorization, using the header property named “Amelia”.
Path
Amelia API paths start with: {{your_site_URL}}/wp-admin/admin-ajax.php?action=wpamelia_api&call=/api/v1
Add package purchase
Create a package purchase.
Method
This endpoint accepts POST requests.
Path
/packages/customers
Required Properties
The following properties are required.
Key | Type | Description |
---|---|---|
packageId | integer | The package id. |
customerId | integer | The customer id. |
notify | boolean | Whether the customer will be notified about this purchase. |
Example
curl --location 'http://localhost/amelia/wp-admin/admin-ajax.php?action=wpamelia_api&call=/api/v1/packages/customers' \ --header 'Content-Type: application/json' \ --header 'Amelia: qUmkNNOLrWbf28izIgNnZ29O+7gVWL5M+8ySJ8VXq3r0' \ --data '{ "packageId": 14, "customerId": 16, "notify": true }'
{ "message": "Successfully added new package booking.", "data": { "packageCustomerId": 115, "notify": true, "paymentId": 1154, "onlyOneEmployee": null } }
Add package appointment
Add an appointment to a package purchase.
Method
This endpoint accepts POST requests.
Path
/appointments
Required Properties
The following properties are required.
Key | Type | Description |
---|---|---|
bookings | array | An array of booking. |
serviceId | integer | The service id. |
providerId | integer | The employee id. |
bookingStart | string | The date and time of the start of the appointment. The format is: “YYYY-MM-DD HH:mm”. |
Optional Properties
Key | Type | Description |
---|---|---|
notifyParticipants
|
integer | Whether the customer should be notified. Possible values: 1, 0. |
internalNotes
|
string | The internal notes of the appointment. |
lessonSpace
|
string | The id of the lesson space room to be used. |
locationId | integer | The location id. |
Example
curl --location 'http://localhost/amelia/wp-admin/admin-ajax.php?action=wpamelia_api&call=/api/v1/appointments' \ --header 'Content-Type: application/json' \ --header 'Amelia: qUmkNNOLrWbf28izIgNnZ29O+7gVWL5M+8ySJ8VXq3r0' \ --data '{ "serviceId": 1, "providerId": 1, "locationId": 2, "bookings": [ { "customerId": 16, "status": "approved", "duration": 3600, "persons": 1, "extras": [], "customFields": "{\"1\":{\"label\":\"text\",\"value\":\"\",\"type\":\"text\"},\"4\":{\"label\":\"Adresa\",\"value\":\"\",\"type\":\"address\"}}", "packageCustomerService": { "packageCustomer": { "id": 115 } } } ], "bookingStart": "2023-11-22 09:30", "notifyParticipants": 1, "internalNotes": "", "lessonSpace": null }'
{ "message": "Successfully added new appointment", "data": { "appointment": { "id": 851, "bookings": [ { "id": 1147, "customerId": 16, "customer": null, "status": "approved", "extras": [], "couponId": null, "price": 23, "coupon": null, "customFields": "{\"1\":{\"label\":\"text\",\"value\":\"\",\"type\":\"text\"},\"4\":{\"label\":\"Adresa\",\"value\":\"\",\"type\":\"address\"}}", "info": null, "appointmentId": 851, "persons": 1, "token": "8c614c916d", "payments": [], "utcOffset": null, "aggregatedPrice": true, "isChangedStatus": true, "isLastBooking": null, "packageCustomerService": { "id": 179, "serviceId": null, "providerId": null, "locationId": null, "bookingsCount": null, "packageCustomer": { "id": 115, "packageId": null, "customerId": null, "price": null, "payments": [], "start": null, "end": null, "purchased": null, "status": null, "bookingsCount": null, "couponId": null, "coupon": null } }, "ticketsData": [], "duration": 3600, "created": null, "actionsCompleted": false, "isUpdated": null }, { "id": 1146, "customerId": 16, "customer": null, "status": "canceled", "extras": [], "couponId": null, "price": 23, "coupon": null, "customFields": "{\"2\":{\"label\":\"Select1\",\"value\":\"\",\"type\":\"select\"},\"5\":{\"label\":\"text3\",\"value\":\"\",\"type\":\"text\"},\"7\":{\"label\":\"My address\",\"value\":\"\",\"type\":\"address\"},\"9\":{\"label\":\"address4\",\"value\":\"\",\"type\":\"address\"},\"13\":{\"label\":\"file\",\"value\":\"\",\"type\":\"file\"}}", "info": "{\"firstName\":\"Amelia\",\"lastName\":\"Test\",\"phone\":\"+381601234567\",\"locale\":null,\"timeZone\":null,\"urlParams\":null}", "appointmentId": 851, "persons": 1, "token": null, "payments": [], "utcOffset": null, "aggregatedPrice": true, "isChangedStatus": null, "isLastBooking": null, "packageCustomerService": { "id": 179, "serviceId": null, "providerId": null, "locationId": null, "bookingsCount": null, "packageCustomer": { "id": null, "packageId": null, "customerId": null, "price": null, "payments": [], "start": null, "end": null, "purchased": null, "status": null, "bookingsCount": null, "couponId": null, "coupon": null } }, "ticketsData": [], "duration": 1800, "created": "2023-11-06 16:33:22", "actionsCompleted": null, "isUpdated": null } ], "notifyParticipants": 0, "internalNotes": null, "status": "approved", "serviceId": 1, "parentId": null, "providerId": 1, "locationId": 2, "provider": null, "service": null, "location": null, "googleCalendarEventId": null, "googleMeetUrl": null, "outlookCalendarEventId": null, "zoomMeeting": null, "lessonSpace": null, "bookingStart": "2023-11-22 09:30:00", "bookingEnd": "2023-11-22 10:30:00", "type": "appointment", "isRescheduled": null, "isFull": null, "resources": [] }, "recurring": [] } }
Update package purchase status
Update package purchase status.
Method
This endpoint accepts POST requests.
Path
/packages/customers/{{package_customer_id}}
Required Properties
The following properties are required.
Key | Type | Description |
---|---|---|
status | string | The new status of the package pucrhase. Possible values: “approved”, “canceled” |
Example
curl --location 'http://localhost/amelia/wp-admin/admin-ajax.php?action=wpamelia_api&call=/api/v1/packages/customers/115' \ --header 'Content-Type: application/json' \ --header 'Amelia: qUmkNNOLrWbf28izIgNnZ29O+7gVWL5M+8ySJ8VXq3r0' \ --data '{ "status": "canceled" }'
{ "message": "Successfully updated package", "data": { "packageCustomerId": "115", "status": "canceled" } }
Get available package slots
Retrieve number of available slots for a package purchase
Method
This endpoint accepts GET requests.
Path
/package-purchases/slots
Optional Properties
You can use the following query parameters for filtering the package purchases.
Key | Type | Description |
---|---|---|
packageId | int | The id of the package |
customerId | int | The id of the customer |
Example
curl --location 'http://localhost/amelia2/wp-admin/admin-ajax.php?action=wpamelia_api&call=/api/v1/package-purchases/slots&packageId=3&customerId=3' \ --header 'Amelia: s7nC4/uAb0dPHnFevx06GKJGryzFxnVeiCwPNmZRD/a+'
{ "message": "Successfully retrieved available package slots", "data": [ { "customerId": 3, "packages": [ { "packageId": 3, "purchases": [ { "purchase": [ { "serviceId": 1, "available": 3, "total": 4 }, { "serviceId": 5, "available": 3, "total": 4 } ], "purchased": "2024-03-29 16:42", "packageCustomerId": 79 } ] } ] } ] }
Delete a package purchase
Delete a package purchase.
Method
This endpoint accepts POST requests.
Path
/packages/customers/delete/{{package_customer_id}}
Example
curl --location --request POST 'http://localhost/amelia/wp-admin/admin-ajax.php?action=wpamelia_api&call=/api/v1/packages/customers/delete/115' \ --header 'Amelia: qUmkNNOLrWbf28izIgNnZ29O+7gVWL5M+8ySJ8VXq3r0' \ --data ''
{ "message": "Successfully deleted package purchase.", "data": { "packageCustomer": { "id": 115, "packageId": 14, "customerId": 16, "price": 180, "payments": [], "start": "2023-11-06 15:25:58", "end": null, "purchased": "2023-11-06 15:25:58", "status": "canceled", "bookingsCount": 0, "couponId": null, "coupon": null }, "appointments": { "updatedAppointments": [ { "appointment": { "id": 851, "bookings": [ { "id": 1146, "customerId": 16, "customer": { "id": 16, "firstName": "Amelia", "lastName": "Test", "birthday": null, "email": "[email protected]", "phone": "+381601234567", "type": "customer", "status": "visible", "note": null, "zoomUserId": null, "countryPhoneIso": null, "externalId": null, "pictureFullPath": null, "pictureThumbPath": null, "translations": null, "gender": null }, "status": "canceled", "extras": [], "couponId": null, "price": 23, "coupon": null, "customFields": "{\"2\":{\"label\":\"Select1\",\"value\":\"\",\"type\":\"select\"},\"5\":{\"label\":\"text3\",\"value\":\"\",\"type\":\"text\"},\"7\":{\"label\":\"My address\",\"value\":\"\",\"type\":\"address\"},\"9\":{\"label\":\"address4\",\"value\":\"\",\"type\":\"address\"},\"13\":{\"label\":\"file\",\"value\":\"\",\"type\":\"file\"}}", "info": "{\"firstName\":\"Amelia\",\"lastName\":\"Test\",\"phone\":\"+381601234567\",\"locale\":null,\"timeZone\":null,\"urlParams\":null}", "appointmentId": 851, "persons": 1, "token": null, "payments": [], "utcOffset": null, "aggregatedPrice": true, "isChangedStatus": false, "isLastBooking": null, "packageCustomerService": { "id": 179, "serviceId": null, "providerId": null, "locationId": null, "bookingsCount": null, "packageCustomer": { "id": null, "packageId": null, "customerId": null, "price": null, "payments": [], "start": null, "end": null, "purchased": null, "status": null, "bookingsCount": null, "couponId": null, "coupon": null } }, "ticketsData": [], "duration": 1800, "created": "2023-11-06 16:33:22", "actionsCompleted": null, "isUpdated": null } ], "notifyParticipants": 0, "internalNotes": null, "status": "canceled", "serviceId": 1, "parentId": null, "providerId": 1, "locationId": 2, "provider": { "id": 1, "firstName": "Milica", "lastName": "Employee", "birthday": null, "email": "[email protected]", "phone": "+381631652656", "type": "provider", "status": null, "note": "note 123345678900", "zoomUserId": null, "countryPhoneIso": null, "externalId": null, "pictureFullPath": null, "pictureThumbPath": null, "translations": null, "weekDayList": [], "serviceList": [], "dayOffList": [], "specialDayList": [], "locationId": null, "googleCalendar": null, "outlookCalendar": null, "timeZone": null, "description": "" }, "service": { "id": 1, "name": "amelia service", "description": "location address: %location_address%", "color": "#1788FB", "price": 20, "deposit": null, "depositPayment": null, "depositPerPerson": null, "pictureFullPath": null, "pictureThumbPath": null, "extras": [], "coupons": [], "position": null, "settings": "{\"payments\":{\"mollie\":{\"enabled\":true},\"paymentLinks\":{\"enabled\":true,\"changeBookingStatus\":false,\"redirectUrl\":null},\"onSite\":true,\"payPal\":{\"enabled\":true},\"stripe\":{\"enabled\":true},\"razorpay\":{\"enabled\":true},\"square\":{\"enabled\":true}},\"zoom\":{\"enabled\":true},\"lessonSpace\":{\"enabled\":true},\"activation\":{\"version\":\"6.7\"},\"general\":{\"minimumTimeRequirementPriorToCanceling\":null}}", "fullPayment": null, "minCapacity": 1, "maxCapacity": 10, "duration": 1800, "timeBefore": 1800, "timeAfter": 1800, "bringingAnyone": null, "show": null, "aggregatedPrice": null, "status": "visible", "categoryId": 1, "category": null, "priority": [], "gallery": [], "recurringCycle": null, "recurringSub": null, "recurringPayment": null, "translations": null, "minSelectedExtras": null, "mandatoryExtra": null, "customPricing": null, "maxExtraPeople": null, "limitPerCustomer": null }, "location": null, "googleCalendarEventId": null, "googleMeetUrl": null, "outlookCalendarEventId": null, "zoomMeeting": { "id": 74364614621, "startUrl": "https://us04web.zoom.us/s/74364614621?zak=eyJ0eXAiOiJKV1QiLCJzdiI6IjAwMDAwMSIsInptX3NrbSI6InptX28ybSIsImFsZyI6IkhTMjU2In0.eyJhdWQiOiJjbGllbnRzbSIsInVpZCI6IlBNUVVXdlVXVEJLMVh6cW11YVM2LUEiLCJpc3MiOiJ3ZWIiLCJzayI6Ijg0OTc4OTYwMzQ5OTI5NjU2NDUiLCJzdHkiOjEwMCwid2NkIjoidXMwNCIsImNsdCI6MCwibW51bSI6Ijc0MzY0NjE0NjIxIiwiZXhwIjoxNjk5MjkyMDcxLCJpYXQiOjE2OTkyODQ4NzEsImFpZCI6Ing4ejhiTG1WUzJLODA0aktOa09lTmciLCJjaWQiOiIifQ.yY74Fe24ABFGBFd775CTVPVB4UPRoLSMBP2CosVpUsw", "joinUrl": "https://us04web.zoom.us/j/74364614621?pwd=YtkRIn36Ct9mwBF6p99ZbQm8sa3bo7.1" }, "lessonSpace": null, "bookingStart": "2023-11-22 09:30:00", "bookingEnd": "2023-11-22 10:30:00", "type": "appointment", "isRescheduled": false, "isFull": null, "resources": [] }, "bookingsWithChangedStatus": [ { "id": 1147, "customerId": 16, "customer": { "id": 16, "firstName": "Amelia", "lastName": "Test", "birthday": null, "email": "[email protected]", "phone": "+381601234567", "type": "customer", "status": "visible", "note": null, "zoomUserId": null, "countryPhoneIso": null, "externalId": null, "pictureFullPath": null, "pictureThumbPath": null, "translations": null, "gender": null }, "status": "rejected", "extras": [], "couponId": null, "price": 23, "coupon": null, "customFields": "{\"1\":{\"label\":\"text\",\"value\":\"\",\"type\":\"text\"},\"4\":{\"label\":\"Adresa\",\"value\":\"\",\"type\":\"address\"}}", "info": null, "appointmentId": 851, "persons": 1, "token": null, "payments": [], "utcOffset": null, "aggregatedPrice": true, "isChangedStatus": true, "isLastBooking": null, "packageCustomerService": { "id": 179, "serviceId": null, "providerId": null, "locationId": null, "bookingsCount": null, "packageCustomer": { "id": null, "packageId": null, "customerId": null, "price": null, "payments": [], "start": null, "end": null, "purchased": null, "status": null, "bookingsCount": null, "couponId": null, "coupon": null } }, "ticketsData": [], "duration": 3600, "created": "2023-11-06 16:34:30", "actionsCompleted": null, "isUpdated": null, "skipNotification": true } ], "bookingDeleted": true, "appointmentDeleted": false, "appointmentStatusChanged": true, "appointmentRescheduled": false, "appointmentEmployeeChanged": null, "appointmentZoomUserChanged": false, "appointmentZoomUsersLicenced": false } ], "deletedAppointments": [] } } }